Flows
Activities can only be used to represent a single action happening on a single channel. Some code could be jumping between channels, for example to move it from the IO channel to the decompression and from there to decoding or other kinds of processing. It’s also possible that a single action is being split up and executed accross different channels, for example in a job based setup.
Flows are used to link multiple actions together. When starting a flow the api will remember the activity currently running on the source channel. Then when the flow is stopped later it will detect which action is running on the new activity channel. In the UI these actions are them linked by a line between them, allowing you to easilly see where an action came from or is splitting off to.
Here’s some example code and how it’s visualized in Qumulus. The flow is used to connect the launch of a new thread to the activity executing inside that thread.
#include <quApi.hpp>
void ThreadRun( quFlowID flowID )
{
qu::ScopedActivityChannel sac( "Thread", true );
qu::ScopedActivity activity( "Do Something" );
std::this_thread::sleep_for( std::chrono::milliseconds( 10 ) );
quStopFlow( flowID, quGetChannelIDForCurrentThread() );
std::this_thread::sleep_for( std::chrono::milliseconds( 100 ) );
}
int main( ... )
{
... Api and Activity Channel initialization
qu::ScopedActivity activity( "Launch Thread" );
std::this_thread::sleep_for( std::chrono::milliseconds( 100 ) );
quFlowID newFlowID = quStartFlow( quGetChannelIDForCurrentThread() );
std::this_thread::sleep_for( std::chrono::milliseconds( 10 ) );
std::thread( std::bind( &ThreadRun, newFlowID ) ).detach();
activity.EndScope();
}
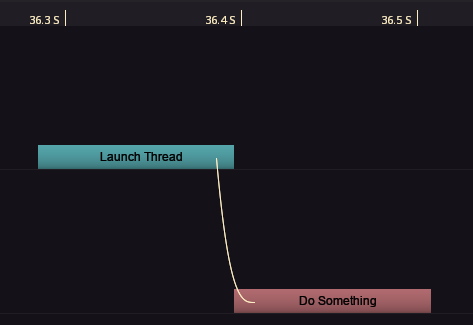